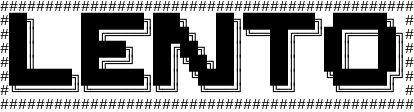
Lento is a highly minimalist and Pythonic package for compiling Lilypond sheet music. The entire API consists of only 2 objects: a class Part
which represents a single instrument, and a main processing function proc
which generates the final outputs (See quick examples below)!
Installation¶
Lento requires Python 3.5 or later:
~$ python --version
Python 3.10.12
Lento requires LilyPond for compiling the final sheet music. Make sure Lilypond is installed and callable from the commandline:
~$ lilypond --version
GNU LilyPond 2.22.2
Lento is on Pypi, so simply use pip to install it:
~$ python -m pip install lento
~$ python -c "import lento; print(lento.version)"
'190624'
First Impressions¶
Start Python, import Lento and print some notes:
from lento import *
proc(Part({"note": range(60, 72), "onset": range(12)}))
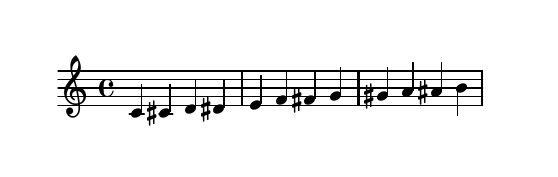
If you want to use two instruments instead of just one, simply pass a list of parts to the processor function:
proc(
[
Part({"note": range(72, 84), "onset": range(12)}),
Part({"note": range(67, 79), "onset": range(12)})
]
)
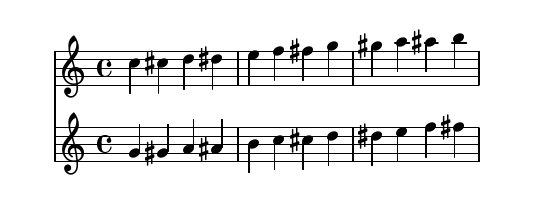
We can also assign specific durations to each onset, if the durations should not be the difference between two neighbor onsets:
from random import choices
ds = choices([pow(x, -1) for x in range(1, 7)], k=12)
print("Durations:", ds)
proc(
[
Part({
"note": range(72, 84),
"onset": range(12),
# Every onset gets the duration of a sixteenth note
"duration": {o: 0.25 for o in range(12)}
}),
Part({
"note": range(67, 79),
"onset": range(12),
# Randomly chosen duration for each onset
"duration": {o: ds[o] for o in range(12)}
})
]
)
Durations: [0.2, 0.25, 0.5, 0.5, 0.2, 0.3333333333333333, 0.2, 1.0, 0.16666666666666666, 1.0, 0.5, 0.3333333333333333]

To have more than one voice in each part, pack multiple pitch and onset collections in a list:
proc(
[
Part({"note": [range(72, 84), range(65, 77)], "onset": [range(12)] * 2}),
Part({"note": [range(60, 72), range(67, 79)], "onset": [range(12)] * 2})
]
)
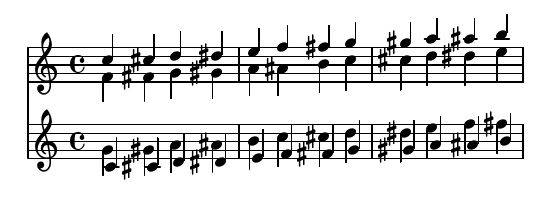
Simple and pythonic!